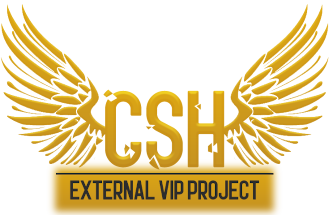
Masz dosyć problemów z czynnikiem zaufania w CS2 lub notorycznymi banami?
Sprawdź CSH External VIP Project.
Więcej informacji
-
Ostatnio przeglądający 0 użytkowników
- Brak zarejestrowanych użytkowników przeglądających tę stronę.
-
Podobna zawartość
Rekomendowane odpowiedzi
Dołącz do dyskusji
Możesz dodać zawartość już teraz a zarejestrować się później. Jeśli posiadasz już konto, zaloguj się aby dodać zawartość za jego pomocą.